Function
Create Datasource / Event
To create a datasource or event using a JavaScript function, follow these steps:
Step 1
Repeat steps 1 and 2 mentioned above to display the creation section and provide a descriptive name for the datasource or event you are creating.
Step 2
Select the “Function” tab.
Step 3
In the “Code Line” field, write the JavaScript function you want to use to fetch the data.
The function has a built-in Axios instance for making HTTP requests that can be used. For example, you can write a function that uses Axios to make a GET request to an API and return or use the retrieved data.
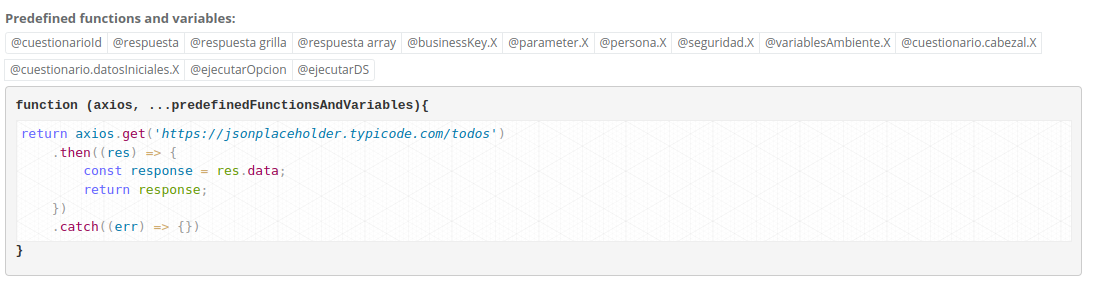
Step 4
Optionally, you can specify the output fields you want to display from the query in the “Out parameters” section below the code line.
Step 5
Next to the name field, you can select the timeout duration.
The timeout is the maximum time it will wait for a response before the request is considered failed. This prevents the request from being blocked indefinitely if the response takes too long.
Step 6
After configuring the JavaScript function and specifying any additional options, you can test the execution in the “Execute” tab. Here, you can see the results of the datasource query and ensure everything works as expected.
Step 7
Finally, save the changes by returning to the “Function” tab and clicking the “Create” button. This will save it with the specified configuration.
Note for Datasources
It is important to know that when defining a function for a datasource, you must ensure that the function returns the query and a resolved promise. This promise should return an array of values to ensure the proper functioning of the datasource.
Example 1
return axios
.get("https://example.com/api/data")
.then((response) => {
return response.data;
})
.catch((error) => {
console.error("Error fetching data:", error);
// Return an empty array in case of an error
return [];
});
Example 2
return [
{ id: 1, name: "Example 1" },
{ id: 2, name: "Example 2" },
{ id: 3, name: "Example 3" },
];